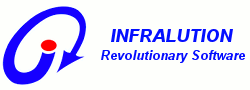 |
Infralution Support Support groups for Infralution products
|
View previous topic :: View next topic |
Author |
Message |
James Guest
|
Posted: Tue Jan 17, 2006 11:06 pm Post subject: Ban certain keys |
|
|
Is it possible to ban certain keys that, for example, may have been posted on a pirate web site? |
|
Back to top |
|
 |
Infralution
Joined: 28 Feb 2005 Posts: 5027
|
Posted: Wed Jan 18, 2006 10:45 pm Post subject: |
|
|
Yes - this is pretty simple. Just check the license SerialNo against a list of known pirated serial numbers and take appropriate action. For instance:
Code: |
License license = null;
if (LicenseManager.IsValid(typeof(MainForm), this, out license))
{
switch ((license as EncryptedLicense).SerialNo)
{
case 45:
case 92:
MessageBox.Show("This is a pirated license key", "Pirated License Key");
// exit the app here
break;
}
}
else
{
// no license installed
} |
_________________ Infralution Support |
|
Back to top |
|
 |
cq Guest
|
Posted: Mon Feb 13, 2006 8:00 pm Post subject: |
|
|
Quote: | License license = null;
if (LicenseManager.IsValid(typeof(MainForm), this, out license))
{
switch ((license as EncryptedLicense).SerialNo)
{
case 45:
case 92:
MessageBox.Show("This is a pirated license key", "Pirated License Key");
// exit the app here
break;
}
}
else
{
// no license installed
} |
Could you please post the VB equivalent?
Thank you. |
|
Back to top |
|
 |
Infralution
Joined: 28 Feb 2005 Posts: 5027
|
Posted: Tue Feb 14, 2006 9:36 am Post subject: |
|
|
It is a little tricker in VB because VB doesn't support unsigned ints (which is what the serial no is). So you need to convert it to a signed Integer before you can do comparisons.
Code: |
Dim sLicense As License = Nothing
If LicenseManager.IsValid(GetType(MyControl), Me, sLicense) Then
Dim serialNo As Integer = Convert.ToInt32(CType(slicense, EncryptedLicense).SerialNo)
Select Case serialNo
Case 45, 92
MessageBox.Show("This is a pirated license key", "Pirated License Key")
' exit the app here
Exit
End Select
End If
|
_________________ Infralution Support |
|
Back to top |
|
 |
cq Guest
|
Posted: Thu Feb 16, 2006 1:49 pm Post subject: |
|
|
Code: |
Dim sLicense As License = Nothing
If LicenseManager.IsValid(GetType(MyControl), Me, sLicense) Then
'more code here
End If
|
Sorry but I cannot understand. Since License = Nothing, IsValid method will always returns False...
How can I fill License instance with appropriate current license? |
|
Back to top |
|
 |
Infralution
Joined: 28 Feb 2005 Posts: 5027
|
Posted: Thu Feb 16, 2006 9:11 pm Post subject: |
|
|
The license parameter of the LicenseManager.IsValid method is an "out" parameter - this is how the LicenseManager passes back the license to you. If you are licensing an application (ie not a control/component) then the latest release of ILS (2.2.0) allows a simpler approach where you don't have to use the LicenseManager class.
' Code: | create the license provider used to get/install the license
'
Dim sProvider As New EncryptedLicenseProvider
Dim sLicense As EncryptedLicense = sProvider.GetLicense(LICENSE_PARAMETERS, LICENSE_FILE)
' check if there is a valid license for the application
'
If sLicense Is Nothing Then
' show install form etc
Else
Dim serialNo As Integer = Convert.ToInt32(sLicense.SerialNo)
Select Case serialNo
Case 45, 92
MessageBox.Show("This is a pirated license key", "Pirated License Key")
' exit the app here
Exit
End Select
End If |
Check out the new samples and documentation for this method. _________________ Infralution Support |
|
Back to top |
|
 |
Guest
|
Posted: Fri Feb 17, 2006 9:15 am Post subject: |
|
|
I'm currently developing a collection of controls.
How can I get LICENSE_FILE? I think I have several LICENSE_FILE's, one for each control. Furthermore, I need a generic method to get sLicense instances of License class.
At the moment, I use this to check the license in the 'ctor:
Code: |
'Licensing
Licensing.EncryptedLicenseProvider.SetParameters(_licenseParameters)
If Not LicenseManager.IsLicensed(Me.GetType()) Then
If LicenseManager.CurrentContext.UsageMode = LicenseUsageMode.Runtime Then
MessageBox.Show("This application was created using an unlicensed version of " & Me.GetType().ToString, "Unlicensed Control")
Else
Dim myForm As New Licensing.LicenseInstallForm
myForm.ShowDialog(Me.GetType().ToString, "www.mycompany.com", Me.GetType())
End If
Else
'This is just to test that license is ok.
MessageBox.Show("Thank you", "Licensed Application")
End If
|
and it seems to works ok.
Later, in the code, I need to check other license informations (ie, SerialNo, ProductInfo, etc.). In order to achieve this, I used this code:
Code: |
Dim sLicense As License = Nothing
If LicenseManager.IsValid(Me.GetType, Me, sLicense) Then
Dim serialNo As Integer = Convert.ToInt32(CType(sLicense, Licensing.EncryptedLicense).SerialNo)
Select Case serialNo
Case 45, 92
MessageBox.Show("This is a pirated license key" & serialNo.ToString, "Pirated License Key")
' exit the app here
Case Else
MessageBox.Show("This is a GOOD license key" & serialNo.ToString, "GOOD License Key")
End Select
Else
MessageBox.Show("License not valid")
End If
|
...but it does not works while sLicense is Nothing.
It is mandatory the use of sProvider.GetLicense(LICENSE_PARAMETERS, LICENSE_FILE)? No alternatives?
Another question. You provided this code:
Code: |
Dim sLicense As License = Nothing
|
Why using License class instead of EncryptedLicense?
Thank you, and sorry for the (apparent) stupidity of my questions. |
|
Back to top |
|
 |
Infralution
Joined: 28 Feb 2005 Posts: 5027
|
Posted: Fri Feb 17, 2006 9:40 am Post subject: |
|
|
If you a licensing controls then you MUST use the LicenseManager methods (ie you should not use the EncryptedLicenseProvider.GetLicense method directly).
If the IsLicensed call works the IsValid should also return true and also return your license information. We use this to license our own controls. Try doing the two calls right next to each other to ensure that you haven't changed something else in between.
Make sure that you have put the LicenseProvider attribute on your control class definition otherwise IsLicensed will always return true even when there is no license and IsValid may return true but no license eg
Code: | <LicenseProvider(GetType(Infralution.Licensing.EncryptedLicenseProvider))> _
Public Class MyControl |
You should also use GetType(MyControl)) rather than Me.GetType(). Me.GetType will return the final type of actual instance. So if someone derives from your control it will return the derived type. Since their derived type is not licensed IsLicensed will again always return true. _________________ Infralution Support |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|